We’ve rolled out several exciting updates to the Admiral Platform designed to streamline your workflows,…
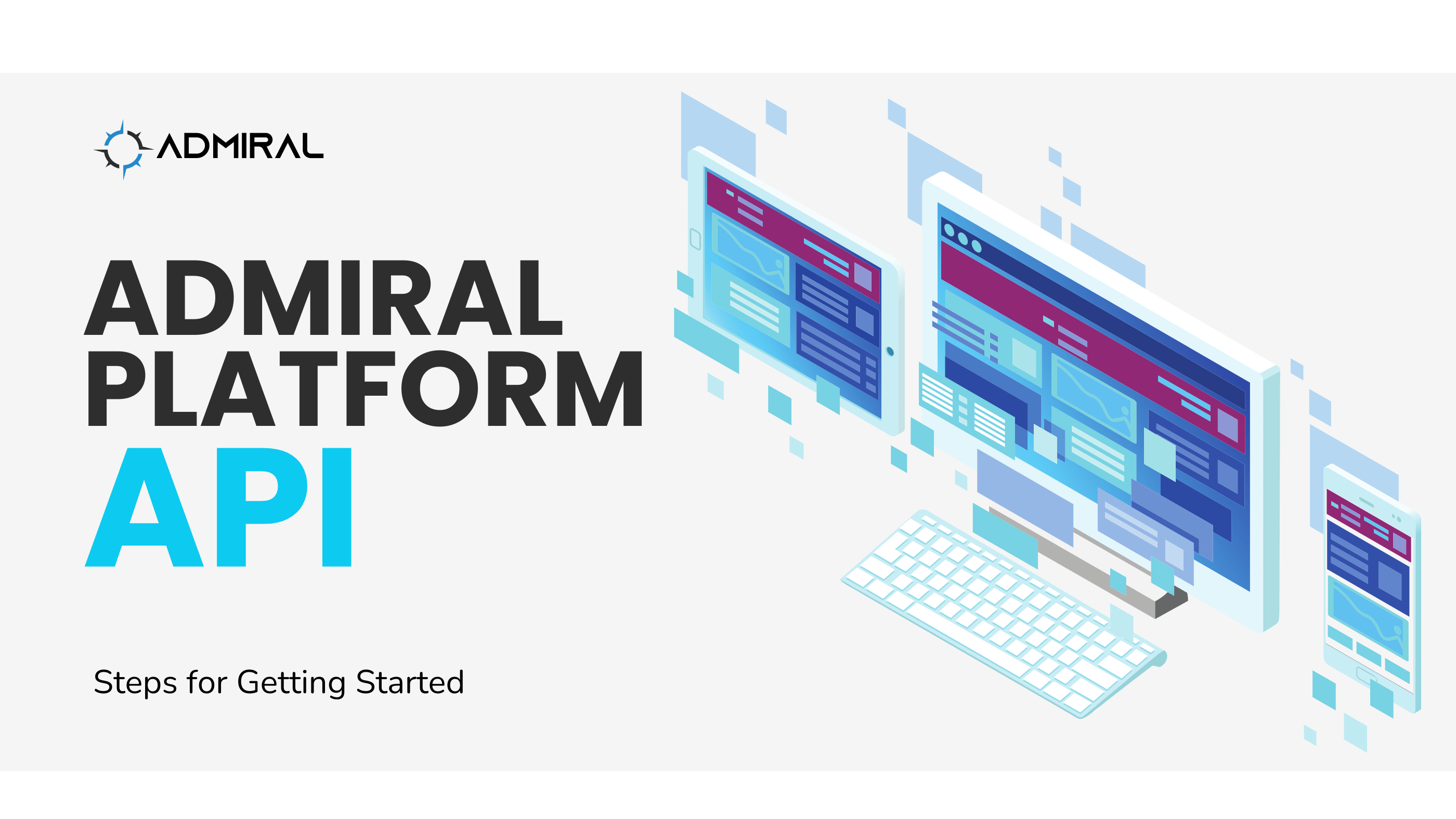
New Feature Release: Admiral Platform API
Coming soon to the Admiral Platform dashboard is a way for network engineers and administrators to integrate Admiral’s powerful tools into their network operations. We will soon be releasing an API to our software, allowing users to further automate their tasks, monitor their networks, and improve their network efficiency.
Getting Started with Admiral Platform API
Follow these steps to start using the Admiral Platform API:
1. Sign Up and Obtain API Credentials
Of course the first step is creating an account with Admiral Platform. If you already have registered for an account, you can navigate to your Profile and find your API Credentials within that page. You will need theses credentials for authenticating your requests.
2. Set Up Your Development Environment
Ensure you have the necessary tools installed. You will need:
- A programming language of your choice (Python, JavaScript, etc.)
- An HTTP client library (requests for Python, Axios for JavaScript)
- A text editor or IDE
- Optional Testing Environment – such as PostMan
3. Authenticate Your Requests
Before making any API calls, you must authenticate your requests using your API credentials. Here’s an example of how to do this in Python using the requests library:
import requests
api_key = 'your_api_key'
api_secret = 'your_api_secret'
base_url = 'https://api.admiralplatform.com'
headers = {
'Authorization': f'Bearer {api_key}:{api_secret}',
'Content-Type': 'application/json'
}
response = requests.get(f'{base_url}/your-endpoint', headers=headers)
if response.status_code == 200:
print('Authenticated successfully!')
else:
print('Authentication failed:', response.text)
4. Explore Available Endpoints
The Admiral Platform API offers various endpoints for different functionalities. Review the API documentation to understand the available endpoints and their parameters. Some common endpoints include:
- /routers – Retrieve a list of all devices
- /router/{id} – Get details of a specific router
PostMan Examples
5.Example API Call
In this example we make a simple API call in Python to fetch a list of devices: (please note this is not an exact example for the API- please follow the documentation within the platform.)
response = requests.get(f'{base_url}/devices', headers=headers)
if response.status_code == 200:
devices = response.json()
for device in devices:
print(f"Device ID: {device['id']}, Name: {device['name']}")
else:
print('Failed to retrieve devices:', response.text)
6. Handle Errors and Responses Example in Python
Properly handling errors and responses is crucial for robust applications. Always check the status code and handle different responses appropriately. Here’s an example: (please note this is not an exact code example for the Admiral API- please follow the documentation within the platform.)
def fetch_devices():
response = requests.get(f'{base_url}/devices', headers=headers)
if response.status_code == 200:
return response.json()
elif response.status_code == 401:
print('Unauthorized: Check your API credentials')
else:
print(f'Error {response.status_code}: {response.text}')
return None
devices = fetch_devices()
if devices:
for device in devices:
print(f"Device ID: {device['id']}, Name: {device['name']}")
Additional Code Examples: (please note this is not an exact code example for the Admiral API- please follow the documentation within the platform.)
PHP – cURL
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://example.remotewinbox.com:8080/auth/apiv1/RWBData/router',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => 'router_name=AdmiralPlatformTest',
CURLOPT_HTTPHEADER => array(
'X-API-KEY: xyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyz',
'Content-Type: application/x-www-form-urlencoded'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
PHP – Guzzle
<?php
$client = new Client();
$headers = [
'X-API-KEY' => 'xyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyz',
'Content-Type' => 'application/x-www-form-urlencoded'
];
$options = [
'form_params' => [
'router_name' => 'AdmiralPlatformTest'
]];
$request = new Request('POST', 'https://example.remotewinbox.com:8080/auth/apiv1/RWBData/router', $headers);
$res = $client->sendAsync($request, $options)->wait();
echo $res->getBody();
JavaScript – jQuery
var settings = {
"url": "https://example.remotewinbox.com:8080/auth/apiv1/RWBData/router",
"method": "POST",
"timeout": 0,
"headers": {
"X-API-KEY": "xyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyz",
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"router_name": "AdmiralPlatformTest"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
Python – Requests
import requests
url = "https://example.remotewinbox.com:8080/auth/apiv1/RWBData/router"
payload = 'router_name=AdmiralPlatformTest'
headers = {
'X-API-KEY': 'xyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyzxyz',
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
7. Integrate with Your Workflow
Once you’re comfortable with the basics, you can integrate the API into your workflow. Automate routine tasks, gather insights through data analysis and build custom applications to enhance your network management capabilities.
Admiral Platform’s API offers a complete solution for network management that’s aligned with your network operations and functions.
Watch for the release of Admiral Platform API to the dashboard within the next couple of weeks and reach out to our support with any comments or questions.
Cheers!